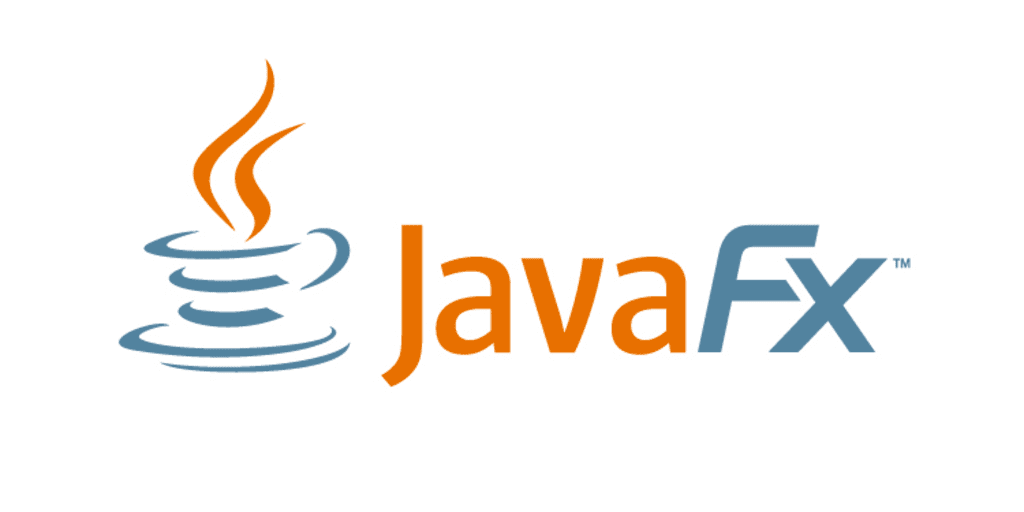
默认样式
先看下表格默认样式:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63
| public class TableViewDemo extends Application {
@Override public void start(Stage stage) {
VBox root = new VBox(); root.setAlignment(Pos.CENTER);
ObservableList<Song> songViews = FXCollections.observableArrayList( );
TableView<Song> tableView = new TableView<>(songViews); tableView.setColumnResizePolicy(TableView.CONSTRAINED_RESIZE_POLICY);
TableColumn<Song, String> nameColumn = new TableColumn<>("标题"); nameColumn.setCellValueFactory(new PropertyValueFactory<>("title")); tableView.getColumns().add(nameColumn);
TableColumn<Song, String> artistColumn = new TableColumn<>("作者"); artistColumn.setCellValueFactory(new PropertyValueFactory<>("artist")); tableView.getColumns().add(artistColumn);
root.getChildren().add(tableView);
Scene scene = new Scene(root, 400, 200); scene.getStylesheets().add(this.getClass().getResource("/css/style.css").toExternalForm()); stage.setScene(scene); stage.setTitle("TableView Demo"); scene.setRoot(root); stage.show(); }
public static void main(String[] args) { launch(args); }
public static class Song { private final SimpleStringProperty title;
private final SimpleStringProperty artist;
public Song(String title, String artist) { this.title = new SimpleStringProperty(title); this.artist = new SimpleStringProperty(artist); }
public String getTitle() { return title.get(); }
public SimpleStringProperty titleProperty() { return title; }
public String getArtist() { return artist.get(); }
public SimpleStringProperty artistProperty() { return artist; } } }
|
效果:
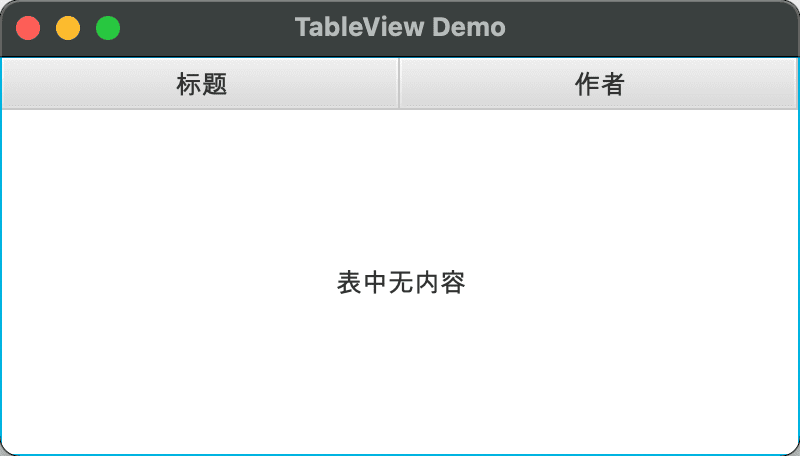
去掉默认文本
当表格中没有内容时,修改文本 表中无内容
:
1 2
| tableView.setPlaceholder(new Text(""));
|
或者可以放张图片:
1
| tableView.setPlaceholder(new ImageView(new Image(new FileInputStream("a.jpg"), 100, 100, false, false)));
|
效果:
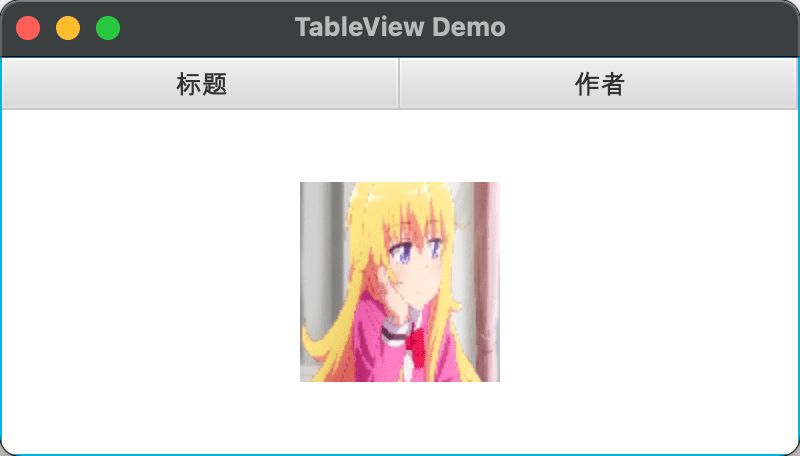
添加索引列
首列展示索引:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| TableColumn<Song, Song> idColumn = new TableColumn<>("#"); idColumn.setSortable(false);
idColumn.setCellValueFactory(param -> new ReadOnlyObjectWrapper<>(param.getValue())); idColumn.setCellFactory(new Callback<TableColumn<Song, Song>, TableCell<Song, Song>>() { @Override public TableCell<Song, Song> call(TableColumn<Song, Song> param) { return new TableCell<Song, Song>() { @Override protected void updateItem(Song item, boolean empty) { super.updateItem(item, empty); if (this.getTableRow() != null && item != null) { setText(String.valueOf(this.getTableRow().getIndex() + 1)); } else { setText(""); } } }; } }); tableView.getColumns().add(idColumn);
|
效果:
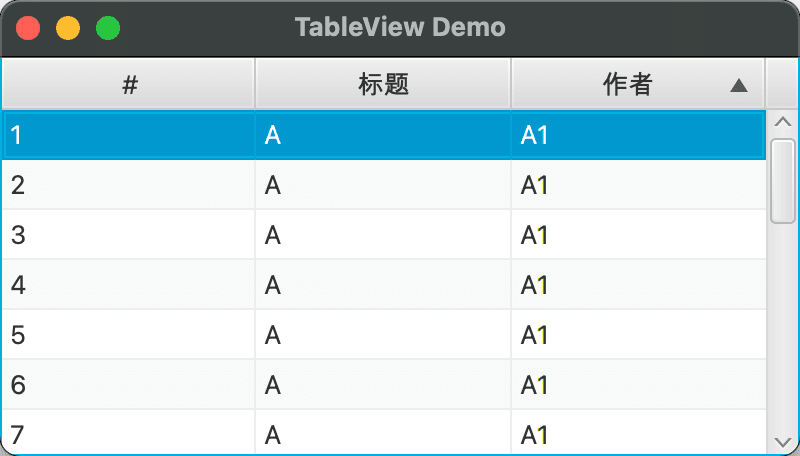
修改行样式
通过修改 .table-row-cell
样式实现,table-row-cell
可以认为是表格中的每一行:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| .table-view .table-row-cell { -fx-text-background-color: white; }
.table-view .table-row-cell:odd { -fx-background-color: green; }
.table-view .table-row-cell:even { -fx-background-color: greenyellow; }
.table-view .table-row-cell:hover { -fx-background-color: white; -fx-text-background-color: black; }
.table-view .table-row-cell:selected { -fx-background-color: red; }
|
效果:
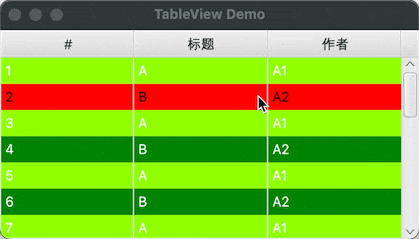
修改列样式
通过修改 .table-column
样式实现,以文本居中为例:
1 2 3
| .table-view .table-column { -fx-alignment: center; }
|
效果:
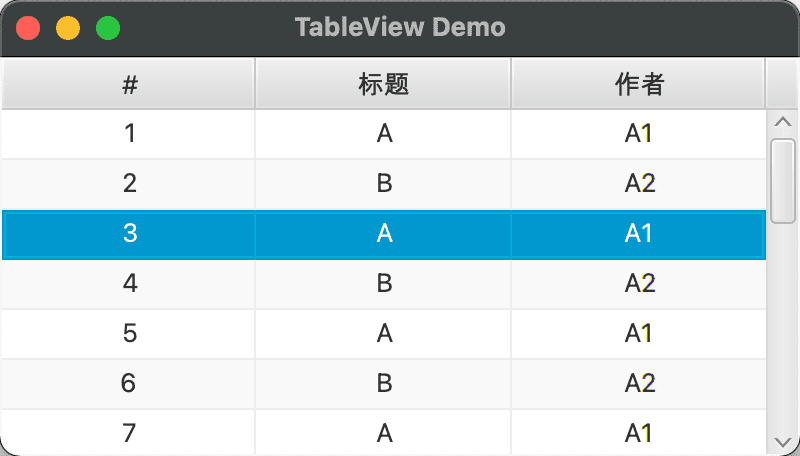
修改单元格样式
通过修改 .table-cell
样式实现,以边框为例:
1 2 3 4
| .table-view .table-cell { -fx-border-color: black; -fx-border-width: 1; }
|
效果:
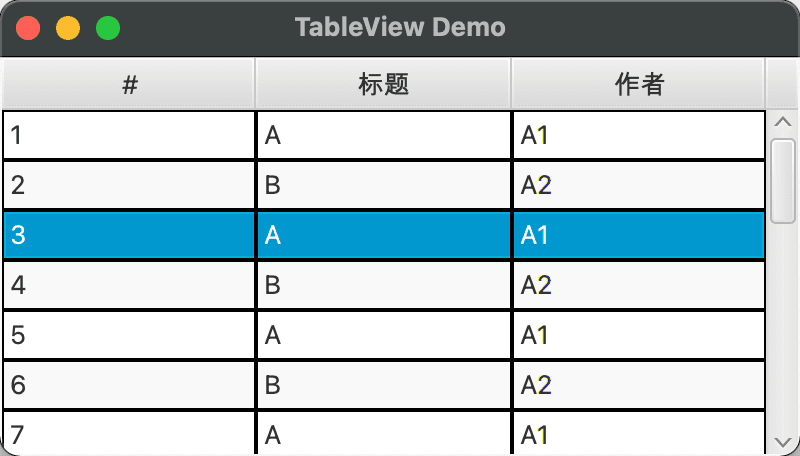
修改表格头样式
通过修改 .column-header
样式实现:
1 2 3 4 5 6 7 8 9 10 11
| .table-view .column-header { -fx-background-color: greenyellow; }
.table-view .column-header-background .label { -fx-font-size: 24; }
.table-view .column-header-background .filler { -fx-background-color: red; }
|
效果:
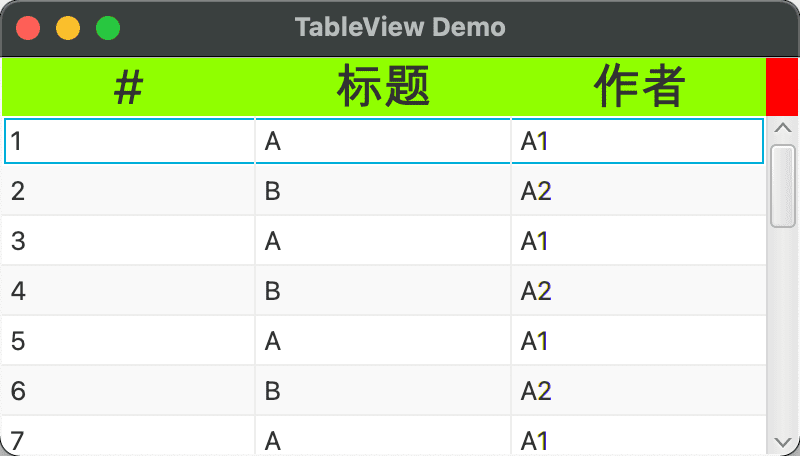
修改滚动条样式
滚动条样式修改方式都类似,如 ScrollBar
,ScrollPane
,TabelView
等。滚动条主要由 Track
,Thumb
,Left Button
,Right Button
组成,此处借用官网介绍图:
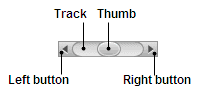
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| .table-view .scroll-bar { -fx-background-color: greenyellow; } .table-view .scroll-bar:vertical .thumb { -fx-background-color: green; } .table-view .scroll-bar:vertical .increment-button, .table-view .scroll-bar:vertical .decrement-button { -fx-background-color: pink; } .table-view .scroll-bar:vertical .increment-arrow, .table-view .scroll-bar:vertical .decrement-arrow { -fx-background-color: red; }
|
效果:
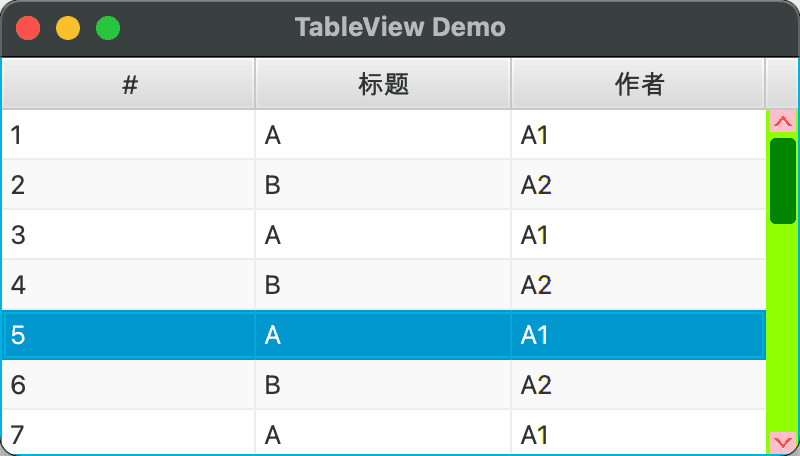
或者可以完全隐藏滚动条:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| .table-view .scroll-bar { -fx-background-color: transparent; -fx-padding: 0; -fx-pref-width: 0; } .table-view .scroll-bar:vertical .thumb { -fx-background-color: transparent; -fx-padding: 0; -fx-pref-width: 0; } .table-view .scroll-bar:vertical .increment-button, .table-view .scroll-bar:vertical .decrement-button, .table-view .scroll-bar:vertical .increment-arrow, .table-view .scroll-bar:vertical .decrement-arrow { -fx-background-color: transparent; -fx-padding: 0; -fx-shape: null; -fx-pref-width: 0; }
|
效果:
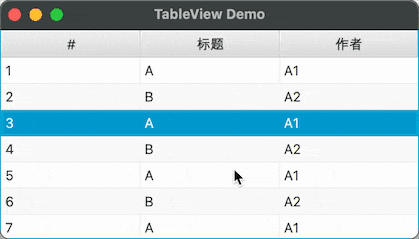
参考
How to style a TableView in JavaFX
JavaFX TableView Column Width\Contents auto-truncating