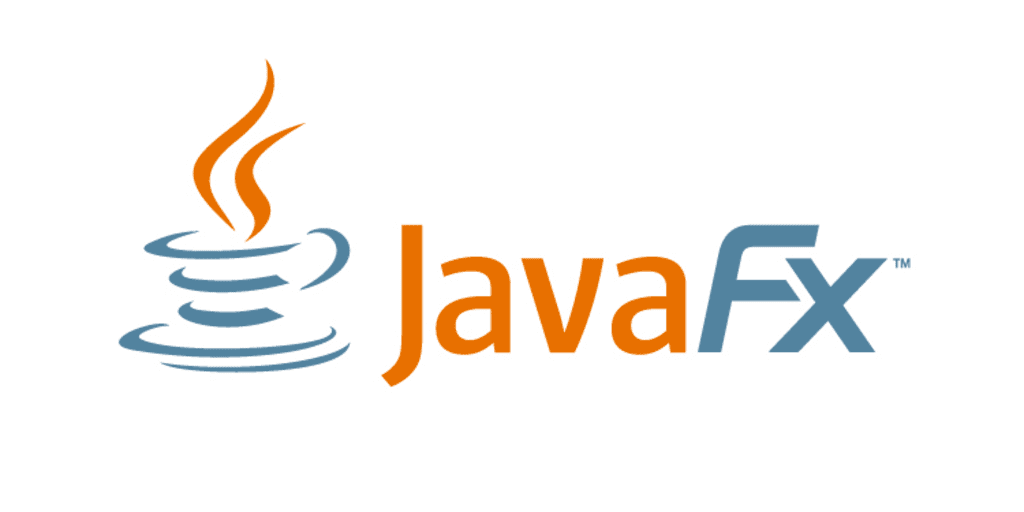
引入外部字体需要注意一点,有些字体只支持英文,因此可能会出现即使设置了字体,但是看不到效果的情况。
本文字体以思源黑体为例,下载地址:https://fonts.google.com/download?family=Noto%20Sans%20SC
默认字体
先看下默认字体效果:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| public class ImportFontDemo extends Application { @Override public void start(Stage stage) {
VBox root = new VBox(); root.setAlignment(Pos.CENTER);
Label text = new Label(); text.setText("这是一段文字"); text.setFont(Font.font(36));
root.getChildren().add(text);
Scene scene = new Scene(root, 400, 200); scene.getStylesheets().add(this.getClass().getResource("/css/style.css").toExternalForm()); stage.setScene(scene); stage.setTitle("Font Demo"); scene.setRoot(root); stage.show(); }
public static void main(String[] args) { launch(args); } }
|
运行效果:
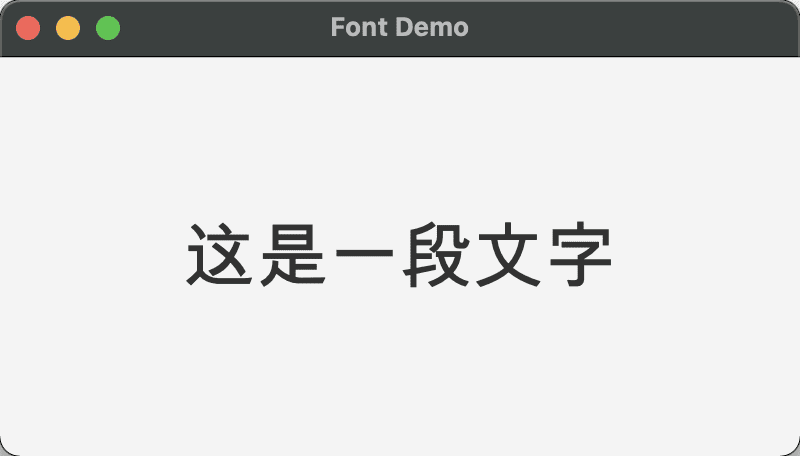
加载本地字体
以 Maven
项目为例,下载字体解压后放到resources/font
文件夹内:
1 2 3 4 5 6 7 8
| - src - main - java - resource - css - font - NotoSansSC-Black.otf - test
|
应用初始化时加载
1 2 3 4 5 6 7 8
| @Override public void init() throws Exception { super.init(); Font font = Font.loadFont(this.getClass().getResource("/font/NotoSansSC-Black.otf").toString(), 18); System.out.println(font); }
|
如果出现字体加载出错,请检查字体文件路径是否正确:
1 2 3 4 5 6 7 8 9 10 11 12 13
| <build> <resources> <resource> <directory>src/main/resources</directory> <includes> <include>css/*</include> <include>font/*</include> </includes> <filtering>false</filtering> </resource> </resources> </build>
|
设置标签字体:
1 2 3
| Label text = new Label(); text.setText("这是一段文字"); text.setFont(Font.font("Noto Sans SC Black", 36));
|
CSS文件中加载
在 CSS
文件中使用:
1 2 3 4 5 6 7
| @font-face { src: url("/font/NotoSansSC-Black.otf"); } .label { -fx-font-family: 'Noto Sans SC Black'; -fx-font-size: 36; }
|
注意:这种方式引入的字体只能在CSS文件中使用
运行效果
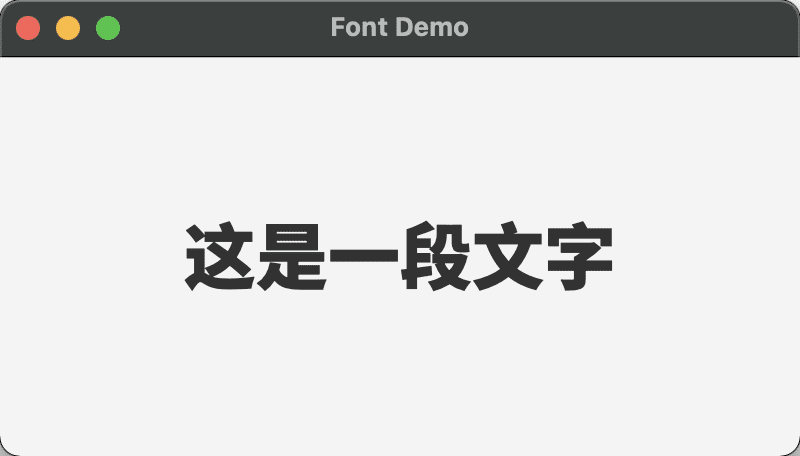
加载在线字体
应用初始化时加载
1 2 3 4 5 6 7
| @Override public void init() throws Exception { super.init(); Font font = Font.loadFont("https://image.iyichen.xyz/NotoSansSC-Thin.otf", 18); System.out.println(font); }
|
设置标签字体:
1 2 3
| Label text = new Label(); text.setText("这是一段文字"); text.setFont(Font.font("Noto Sans SC Thin", 36));
|
引入CSS文件时加载
1 2 3
| scene.getStylesheets().add("https://fonts.googleapis.com/css2?family=Noto+Sans+SC:wght@100&display=swap"); scene.getStylesheets().add(this.getClass().getResource("/css/style.css").toExternalForm());
|
或者:
1 2 3
| @font-face { src: url("https://image.iyichen.xyz/NotoSansSC-Thin.otf"); }
|
注意:以上两种方式引入的字体只能在CSS文件中使用
运行效果
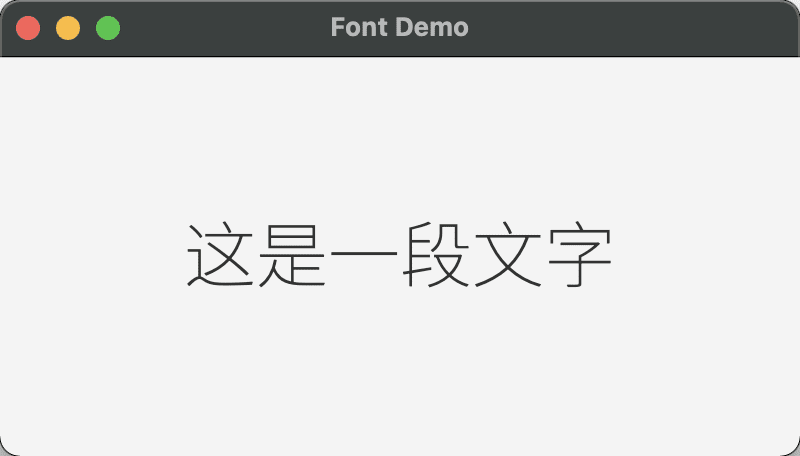